Gesture Controlled Robot Car With Accelerometer
Engineer High School
Ilan Goldfein SAR High School
Area of Interest Grade
Software Engineering Incoming Freshman
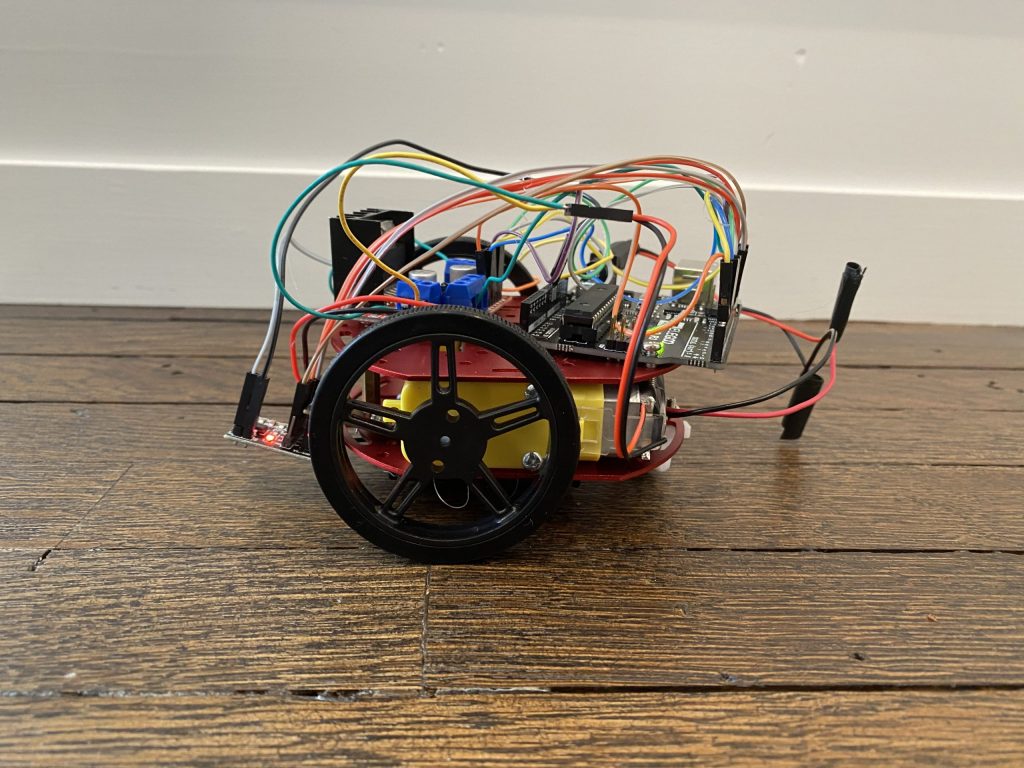
Milestone Three
My third Milestone was to present my project and add the finishing touches. First, I added all the controller circuits onto a glove for simpler control. Next, I needed to fit all of the car hardware onto the chassis. Because of the small size of the chassis, this was not so easy. I hot glued the motor driver battery pack to the bottom of the car to make space for the arduino. Next, I took a 9v battery clip and soldered it to a 9V DC plug so I would be able to power the arduino. That battery then went in the middle of the motors. I then screwed the arduino and the motor driver into the top of the car to keep them from falling off.
Photos
Milestone two
My second Milestone was to finish my base project. I began doing that by sending the accelerometer values to the robot. I was able to accomplish this by using nRF modules. Using these modules was not easy. To do this, I created a sendData() function and then passed direction values as character arrays into that function. The function would then take those values and send them over nRF to the robot. Once the robot had those values, I then had it map them into the different directions. I mapped the directions by creating if statements for the different xyz values. Once the robot had those directions, it then sent them to the motor driver to move the car.
Wiring
#include <SPI.h> //SPI library for communicate with the nRF24L01+ #include "RF24.h" //The main library of the nRF24L01+ #include "Wire.h" //For communicate #include "I2Cdev.h" //For communicate with MPU6050 #include "MPU6050.h" //The main library of the MPU6050 //Define the object to access and cotrol the Gyro and Accelerometer (We don't use the Gyro data) MPU6050 mpu; int16_t ax, ay, az; int16_t gx, gy, gz; //Define packet for the direction (X axis and Y axis) int data[2]; //Define object from RF24 library - 9 and 10 are a digital pin numbers to which signals CE and CSN are connected. RF24 radio(9,10); //Create a pipe addresses for the communicate const uint64_t pipe = 0xE8E8F0F0E1LL; void setup(void){ Serial.begin(9600); Wire.begin(); mpu.initialize(); //Initialize the MPU object radio.begin(); //Start the nRF24 communicate radio.openWritingPipe(pipe); //Sets the address of the receiver to which the program will send data. } void loop(void){ //With this function, the acceleration and gyro values of the axes are taken. //If you want to control the car axis differently, you can change the axis name in the map command. mpu.getMotion6(&ax, &ay, &az, &gx, &gy, &gz); //In two-way control, the X axis (data [0]) of the MPU6050 allows the robot to move forward and backward. //Y axis (data [0]) allows the robot to right and left turn. data[0] = map(ax, -17000, 17000, 300, 400 ); //Send X axis data data[1] = map(ay, -17000, 17000, 100, 200); //Send Y axis data radio.write(data, sizeof(data)); }
#include <SPI.h> //SPI library for communicate with the nRF24L01+ #include "RF24.h" //The main library of the nRF24L01+ //Define enable pins of the Motors const int enbA = 3; const int enbB = 5; //Define control pins of the Motors //If the motors rotate in the opposite direction, you can change the positions of the following pin numbers const int IN1 = 2; //Right Motor (-) const int IN2 = 4; //Right Motor (+) const int IN3 = 7; //Left Motor (+) const int IN4 = 6; //Right Motor (-) //Define variable for the motors speeds //I have defined a variable for each of the two motors //This way you can synchronize the rotation speed difference between the two motors int RightSpd = 130; int LeftSpd = 150; //Define packet for the direction (X axis and Y axis) int data[2]; //Define object from RF24 library - 9 and 10 are a digital pin numbers to which signals CE and CSN are connected RF24 radio(9,10); //Create a pipe addresses for the communicate const uint64_t pipe = 0xE8E8F0F0E1LL; void setup(){ //Define the motor pins as OUTPUT pinMode(enbA, OUTPUT); pinMode(enbB, OUTPUT); pinMode(IN1, OUTPUT); pinMode(IN2, OUTPUT); pinMode(IN3, OUTPUT); pinMode(IN4, OUTPUT); Serial.begin(9600); radio.begin(); //Start the nRF24 communicate radio.openReadingPipe(1, pipe); //Sets the address of the transmitter to which the program will receive data. radio.startListening(); } void loop(){ if (radio.available()){ radio.read(data, sizeof(data)); if(data[0] > 380){ //forward analogWrite(enbA, RightSpd); analogWrite(enbB, LeftSpd); digitalWrite(IN1, HIGH); digitalWrite(IN2, LOW); digitalWrite(IN3, HIGH); digitalWrite(IN4, LOW); } if(data[0] < 310){ //backward analogWrite(enbA, RightSpd); analogWrite(enbB, LeftSpd); digitalWrite(IN1, LOW); digitalWrite(IN2, HIGH); digitalWrite(IN3, LOW); digitalWrite(IN4, HIGH); } if(data[1] > 180){ //left analogWrite(enbA, RightSpd); analogWrite(enbB, LeftSpd); digitalWrite(IN1, HIGH); digitalWrite(IN2, LOW); digitalWrite(IN3, LOW); digitalWrite(IN4, HIGH); } if(data[1] < 110){ //right analogWrite(enbA, RightSpd); analogWrite(enbB, LeftSpd); digitalWrite(IN1, LOW); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, LOW); } if(data[0] > 330 && data[0] < 360 && data[1] > 130 && data[1] < 160){ //stop car analogWrite(enbA, 0); analogWrite(enbB, 0); } } }
Milestone one
Example of the accelerometer 3D mapping with processing
My First Milestone was to have the basic components of the project working. The first thing I needed to get working was the motors. I used an H-Bridge to program simple directional control. Next, I used the accelerometer module to receive the hand-tilt data in XYZ format. I was then able to use that data in two ways. 1. I used the serial monitor to see the yaw, pitch, and roll values, which I will soon change into left, right, forward, etc. 2. I used processing to 3d map the data to make sure that I was receiving the correct values.